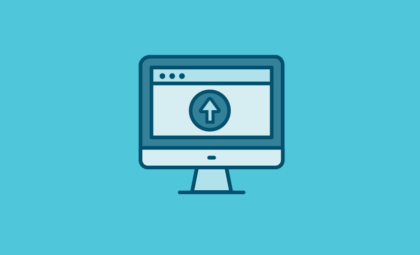
Adding a sticky back-to-top button to your website adds further ease of navigation to your website by allowing a user to scroll to the top of any given web page with the click of a button.
What is a sticky back-to-top button?
Also known as a scroll-to-top button or go-to-top image, the sticky back-to-top button is a helpful navigation element that helps users get back to the top of the web page they’re viewing. A common UI pattern is to place this button in the lower right-hand corner of the screen, making it “sticky” via a fixed position so it’s always accessible as the user scrolls down the page.
How to add a sticky back-to-top button to your WordPress site
In this tutorial, I’ll show you how to add the exact back-to-top button we use here on Layout!
Pro-tip: While this tutorial isn’t too advanced, I still recommend trying it out on a staging site or local environment, so there’s absolutely no risk to your live site.
For this sticky back-to-top button tutorial, we’ll use three languages:
- HTML for the markup to create the button
- CSS to style the button and have it match your brand
- JavaScript to make it “work” and define the behaviors of the button
The HTML we’ll need is extremely simple: just a button element. Go ahead and open your WordPress (child) theme’s footer.php file and right before the body element closes add the following: footer.php
<button class="button-top">↑</button>
Of course, the icon is up to you, I chose a simple up arrow entity for brevity.
If we refresh the page right now we should see an ugly button sitting below our website’s footer, so let’s give it some style; in our (child) theme’s stylesheet: style.css
.button-top {
position: fixed;
bottom: 20px;
right: 20px;
z-index: 100;
width: 60px;
height: 60px;
border: 0;
border-radius: 2px;
box-shadow: none;
background: #145474;
color: #fff;
font-size: 26px;
line-height: 20px;
text-align: center;
cursor: pointer;
}
Pretty cool so far, but before we go any further let’s add a few more styles. We want to start by having the button hidden, and then we’ll define a new class which we’ll later enable via JavaScript and turn it back to visible: style.css
.button-top {
...previous code
pointer-events: none;
opacity: 0;
transition: opacity .18s ease;
}
.button-top-visible {
opacity: 1;
pointer-events: auto;
}
Making it functional
All righty, we’ve got our button styled and ready (and invisible), time to actually make it work. The first thing we need to do is to actually make it smooth scroll back to the top when it’s clicked. Let’s add that code in our theme’s scripts file (make sure jQuery is loaded!): scripts.js
jQuery(function ($) {
var $buttonTop = $('.button-top');
$buttonTop.on('click', function () {
$('html, body').animate({
scrollTop: 0,
}, 400);
});
});
Now every time the button is clicked, it’ll scroll us to the very top of our website within 400 milliseconds (feel free to adjust the timing to your liking).
If we hadn’t required that the button starts hidden we’d be done right now. But we want to show it only when the user has scrolled enough to actually be useful.
What we need for this is to tap into the scroll event of the browser’s window object and check if we’re scrolled enough from the top; if we are, we’ll add that .button-top-visible CSS class and show it. If we aren’t we’ll remove it (and consequently hide the button).
So, the actual event we’re after is probably the scroll event as the name implies, but how are we going to figure out how much we’ve scrolled so far? Let’s see what jQuery offers.
Well, that sounds exactly what we need. Let’s amend our code and do just that: scripts.js
jQuery(function ($) {
var $window = $(window);
var $buttonTop = $('.button-top');
$buttonTop.on('click', function () {
$('html, body').animate({
scrollTop: 0,
}, 400);
});
$window.on('scroll', function () {
if ($window.scrollTop() > 100) { // 100 is our threshold in pixels
$buttonTop.addClass('button-top-visible');
} else {
$buttonTop.removeClass('button-top-visible');
}
});
});
Perfect! Inside our scroll event listener, we check for a simple condition, if we’re scrolled more than 100 pixels we’ll show the button, if not, we’ll hide it!
And that’s it! We’re ready to add a simple back-to-top button to our WordPress (or any kind of) website! Feel free to post in the comments if you decide to add it on your own website or have already done so!